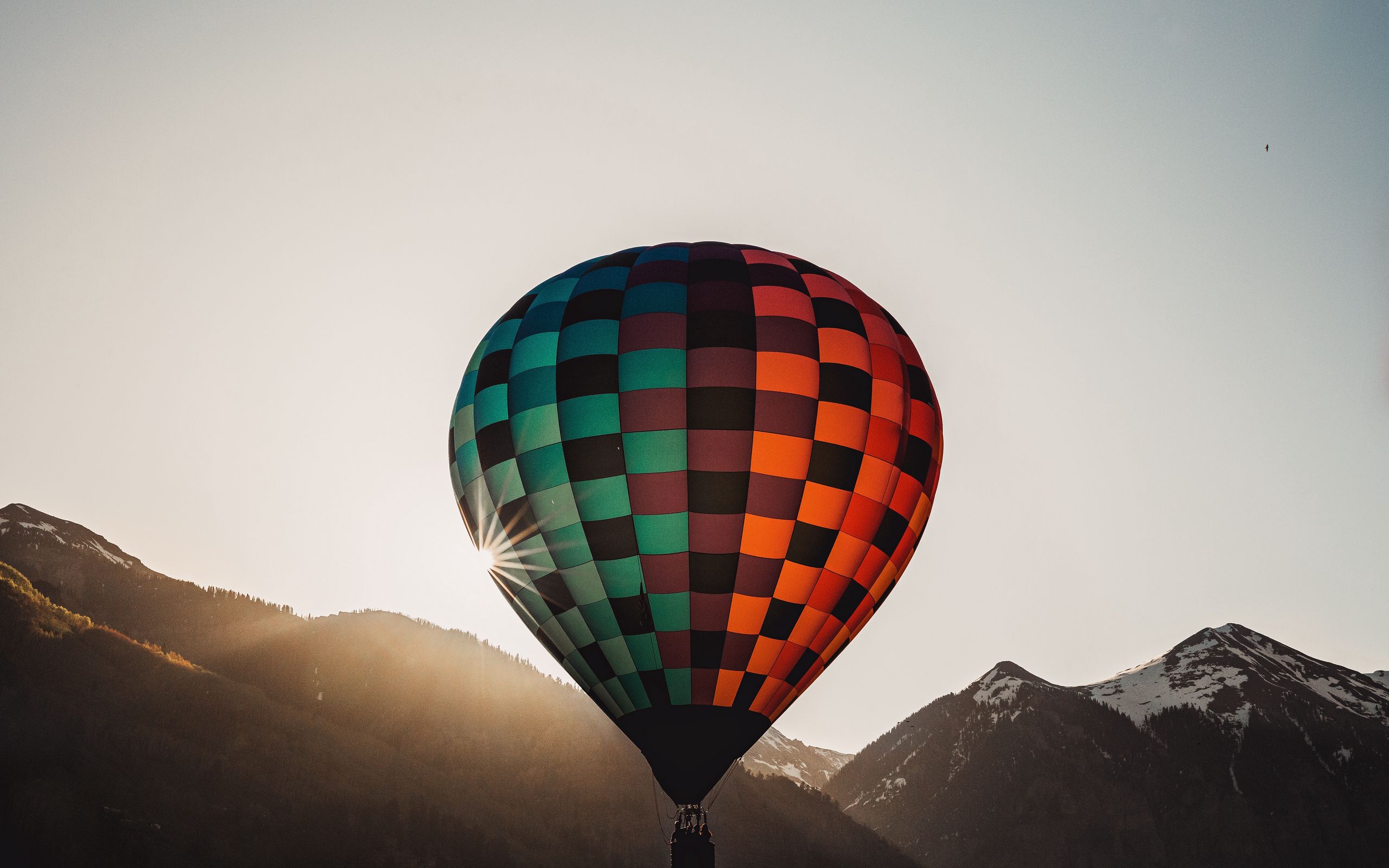
【Pandas+Pyecharts】电商订单数据可视化
【Pandas+Pyecharts】电商订单数据可视化
·
import pandas as pd
from pyecharts.charts import Scatter
from pyecharts.charts import Polar
from pyecharts.charts import Map
from pyecharts import options as opts
from pyecharts.commons.utils import JsCode
from pyecharts.globals import ThemeType
- 基本数据处理
df = pd.read_excel('天猫订单.xlsx')
df.head(3)
订单创建时间 | 订单付款时间 | 订单金额 | 实付金额 | 退款金额 | 收货地址 | |
---|---|---|---|---|---|---|
0 | 2020-02-01 00:14:00 | 2020-02-01 00:14:00 | 38.0 | 0.0 | 38.0 | 四川省 |
1 | 2020-02-01 00:17:00 | 2020-02-01 00:17:00 | 38.0 | 38.0 | 0.0 | 江苏省 |
2 | 2020-02-01 00:33:00 | 2020-02-01 00:33:00 | 76.0 | 0.0 | 76.0 | 湖北省 |
df.info()
<class 'pandas.core.frame.DataFrame'>
RangeIndex: 28010 entries, 0 to 28009
Data columns (total 6 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 订单创建时间 28010 non-null datetime64[ns]
1 订单付款时间 24087 non-null datetime64[ns]
2 订单金额 28010 non-null float64
3 实付金额 28010 non-null float64
4 退款金额 28010 non-null float64
5 收货地址 28010 non-null object
dtypes: datetime64[ns](2), float64(3), object(1)
memory usage: 1.3+ MB
- 未完成订单
df[df['订单付款时间'].isnull()].head(3)
订单创建时间 | 订单付款时间 | 订单金额 | 实付金额 | 退款金额 | 收货地址 | |
---|---|---|---|---|---|---|
13 | 2020-02-01 07:20:00 | NaT | 76.0 | 0.0 | 0.0 | 广东省 |
17 | 2020-02-01 08:33:00 | NaT | 38.0 | 0.0 | 0.0 | 辽宁省 |
18 | 2020-02-01 08:51:00 | NaT | 114.0 | 0.0 | 0.0 | 重庆 |
-
部分订单创建后没有付款是正常现象
-
时间列都是datetime64格式, 金额列都是float64格式
-
这里只保留付款了的订单
-
添加星期列
#df = df[~df['订单体坛时间'].isnull()]
df['星期'] = df['订单创建时间'].dt.dayofweek+1
df.head(3)
订单创建时间 | 订单付款时间 | 订单金额 | 实付金额 | 退款金额 | 收货地址 | 星期 | |
---|---|---|---|---|---|---|---|
0 | 2020-02-01 00:14:00 | 2020-02-01 00:14:00 | 38.0 | 0.0 | 38.0 | 四川省 | 6 |
1 | 2020-02-01 00:17:00 | 2020-02-01 00:17:00 | 38.0 | 38.0 | 0.0 | 江苏省 | 6 |
2 | 2020-02-01 00:33:00 | 2020-02-01 00:33:00 | 76.0 | 0.0 | 76.0 | 湖北省 | 6 |
df['星期'].unique()
array([6, 7, 1, 2, 3, 4, 5], dtype=int64)
1.一周每天各时段订单数量散点图
- 解析一下下面的 to_fr点e(‘数量’) 的用法
- df.groupby([df[‘星期’],df[‘订单创建时间’].dt.hour])[‘订单创建时间’].count() 这部分生成的是一个两级索引的Series
- to_fr点e(‘数量’) 里的 “数量” 用于传递进去替换原有列名(如果有)
hours = ['0点', '1点', '2点', '3点', '4点', '5点', '6点', '7点', '8点', '9点','10点','11点','12点', '13点', '14点', '15点', '16点', '17点', '18点', '19点', '20点', '21点', '22点', '23点']
weeks = ['周一', '周二', '周三', '周四', '周五', '周六', '周日']
week_hour_group = df.groupby([df['星期'],df['订单创建时间'].dt.hour])['订单创建时间'].count().to_frame('数量')
count_values = list(week_hour_group['数量'].values)
allinfo = []
for idx, dt in enumerate(week_hour_group.index.to_list()):
info = [dt[0], dt[1], count_values[idx]]
allinfo.append(info)
- 下面用另一种方法实现 Datefr点e 整体转为每行做为一个list的list
week_hour_group.reset_index(inplace=True)
allinfo_1 = []
for i in week_hour_group.index:
info_1 = week_hour_group.iloc[i,:].tolist()
allinfo_1.append(info_1)
scatter = (
Scatter(
init_opts=opts.InitOpts(
width='1080px',
height='800px',
theme='light',
bg_color='#0d0735'
)
)
.add_xaxis(xaxis_data=hours)
.set_global_opts(
xaxis_opts=opts.AxisOpts(
is_show=False,
),
yaxis_opts=opts.AxisOpts(
is_show=False
)
)
)
single_axis, titles = [], []
for idx, day in enumerate(weeks[::-1]):
scatter.add_xaxis(xaxis_data=hours)
# 单轴配置
single_axis.append({'left': 100,
'n点eGap': 20,
'n点eLocation': 'start',
'type': 'category',
'boundaryGap': False,
'data': hours,
'top': '{}%'.format(idx * 100 / 7 + 5),
'height': '{}%'.format(100 / 7 - 10),
'gridIndex': idx,
'axisLabel': {'interval': 2,'color':'#9FC131'},
})
titles.append(dict(text=day,top='{}%'.format(idx * 100 / 7 + 6), left='2%',
textStyle=dict(color='#fff200')))
scatter.add_yaxis('',
y_axis=[int(item[2]) for item in allinfo if item[0] == 7-idx],
symbol_size=JsCode('function(p) { return p[1] * 0.15;}'),
label_opts=opts.LabelOpts(is_show=False),
)
scatter.options['series'][idx]['coordinateSystem'] = 'singleAxis'
scatter.options['series'][idx]['singleAxisIndex'] = idx
# 多标题配置
scatter.options['singleAxis'] = single_axis
scatter.set_global_opts(
title_opts=titles
)
scatter.render_notebook()
2.一周各天订单数量极坐标图
week_group = df.groupby(df['星期'])['订单创建时间'].count().to_frame('数量')
polar = (
Polar(
init_opts=opts.InitOpts(
width='1000px',
height='800px',
bg_color='#0d0735'
)
)
.add_schema(
radiusaxis_opts=opts.RadiusAxisOpts(
data=weeks,
type_='category',
axislabel_opts=opts.LabelOpts(
font_size=14,
color='#00aeff'
),
axisline_opts=opts.AxisLineOpts(
is_show=True,
linestyle_opts=opts.LineStyleOpts(
width=2,
color='#9FC131'
)
)
),
angleaxis_opts=opts.AngleAxisOpts(
is_clockwise=True,
is_scale=True,
max_=5500,
axislabel_opts=opts.LabelOpts(
font_size=14,
color='#3be8b0'
),
axisline_opts=opts.AxisLineOpts(
is_show=True,
linestyle_opts=opts.LineStyleOpts(
width=2,
type_='dashed',
color='#e4e932'
)
),
splitline_opts=opts.SplitLineOpts(
is_show=True,
linestyle_opts=opts.LineStyleOpts(
type_='dashed',
color='#9FC131'
)
)
)
)
.add(
'',
week_group['数量'].values.tolist(),
type_='bar'
)
.set_global_opts(
title_opts=opts.TitleOpts(
title='一周各天订单数量分布',
subtitle='▲▼●◆☺☹☀♥☑▓〓',
pos_left='center',
pos_top='1%',
title_textstyle_opts=opts.TextStyleOpts(
color='#fff200',
font_size=20
)
),
visualmap_opts=opts.VisualMapOpts(
max_=6000,
is_show=False,
is_piecewise=True,
split_number=8,
min_=2500,
range_color=[
'#fff7f3',
'#fde0dd',
'#fcc5c0',
'#fa9fb5',
'#f768a1',
'#dd3497',
'#ae017e',
'#7a0177'
]
)
)
)
polar.render_notebook()
3.天猫订单全国地图分布
df_tmp = df.replace('省', '', regex=True).replace('市', '', regex=True).replace('自治区', '', regex=True).replace('壮族', '', regex=True).replace('维吾尔', '', regex=True).replace('回族', '', regex=True)
area_group = df_tmp.groupby('收货地址')['订单创建时间'].count().to_frame('数量')
color_js = """new echarts.graphic.LinearGradient(0, 0, 1, 0,
[{offset: 0, color: '#009ad6'}, {offset: 1, color: '#ed1941'}], false)"""
map = (
Map(
init_opts=opts.InitOpts(
theme='dark',
width='1000px',
height='600px',
bg_color='#0d0735'
)
)
.add(
'',
[list(z) for z in zip(area_group.index.tolist(), area_group['数量'].tolist())],
maptype='china',
is_map_symbol_show=False,
label_opts=opts.LabelOpts(
is_show=True,
color='red'
),
itemstyle_opts={
'normal':{
'shadowColor':'rgba(0,0,0,.5)', #阴影颜色
'shadowBlur':5, #阴影大小
'shadowOffsetY':0, #Y轴方向阴影偏移
'shadowOffsetX':0, #X轴方向阴影偏移
'borderColor':'#fff'
}
}
)
.set_global_opts(
visualmap_opts=opts.VisualMapOpts(
is_show=True,
is_piecewise=True,
min_=0,
max_=3500,
split_number=7,
series_index=0,
pos_top='70%',
pos_left='10%',
range_color=['#9ecae1','#6baed6','#4292c6','#2171b5','#08519c','#08306b','#d4b9da','#c994c7','#df65b0','#e7298a','#ce1256','#980043','#67001f']
),
tooltip_opts=opts.TooltipOpts(
is_show=True,
trigger='item',
formatter='{b}: {c}'
),
title_opts=opts.TitleOpts(
title='天猫订单全国地图分布',
subtitle='▲▼●◆☺☹☀♥☑▓〓',
pos_left='center',
pos_top='5%',
title_textstyle_opts=opts.TextStyleOpts(
color='#fff200',
font_size=20
)
)
)
)
map.render_notebook()
更多推荐
所有评论(0)